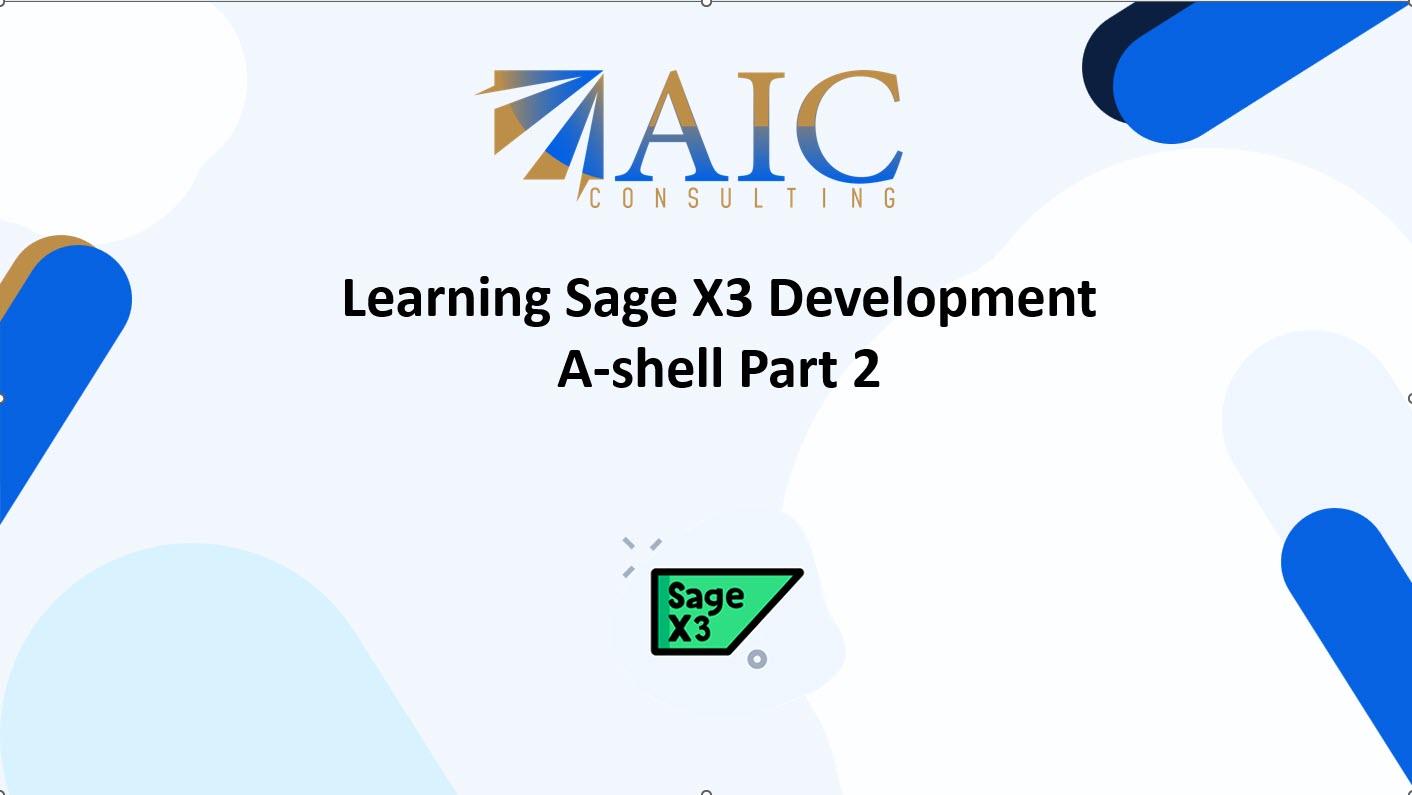
Learning Sage X3 A-shell Development
We’ll cover the fundamentals of Learning Sage X3 Development coding language (A-shell or 4GL).
Let’s dive into the fascinating world of A-shell, the scripting language that brings Sage X3 to life!
Think of A-shell as the language you use to communicate with Sage X3. Just like English helps you chat with your friends, A-shell helps you tell Sage X3 what to do. It’s all about giving instructions in a way Sage X3 understands.
Learning Sage X3 A-shell Development: Syntax and Data Structures
Syntax is like the grammar of A-shell. It’s the set of rules that dictate how you write your code. Just like English has rules about sentence structure, A-shell has rules about how you combine words and symbols to create meaningful instructions.
For example, in A-shell, you might use keywords like If, Then, Else, For, and While to control the flow of your code. These are like the action verbs in English, telling Sage X3 what actions to perform.
Data structures are like containers for storing information. Imagine you’re organizing a kitchen. You might use different containers for storing different ingredients – a jar for spices, a bowl for fruits, and a box for vegetables. Similarly, in A-shell, you use data structures like variables, arrays, and objects to store different types of data.
Here’s a simple example:
#Declare a variable named “zname” and store the value “Alice” in it
Local Char zname : zname = “Alice”
#Display the value of the variable “zname”
In this code snippet, zname is a variable (a container), and “Alice” is the data (the ingredient) stored in that container. The infbox command is an instruction (like an action verb) that tells Sage X3 to display the value of the variable.
Now, let’s see if you’re following along. What do you think would happen if you tried to run the following code?
Local Interger zage : zage = 25
Infbox num$(zage)
Answer : 25
Operators
Let’s explore some other essential elements of A-shell syntax that will empower you to write more sophisticated and effective code.
Operators are like the verbs and adjectives of A-shell, allowing you to perform actions and make comparisons. Think of them as the tools in your kitchen that help you prepare your ingredients.
Here are a few common types of operators:
Arithmetic Operators: These help you perform mathematical calculations, like adding (+), subtracting (-), multiplying (*), and dividing (/). Just like a chef uses measuring cups and scales to get the right proportions, you use arithmetic operators to manipulate numbers in your code.
Comparison Operators: These help you compare values, like checking if two things are equal (=), not equal (<>), greater than (>), or less than (<). It’s like a chef tasting two sauces to see which one is spicier.
Logical Operators: These help you combine or modify conditions, like checking if two things are both true (and), if at least one of two things is true (or), or if something is not true (not). This is like a chef deciding whether a dish needs both salt and pepper, or just one of them.
Comments are like little notes you leave in your code to explain what’s going on. They’re ignored by A-shell, but they’re super helpful for you and other programmers who might read your code later. It’s like a chef writing down the steps of a recipe so they can remember it next time. In A-shell, you can add a comment by starting a line with a #. Everything after the # on that line will be treated as a comment.
Here’s an example: # This code calculates the area of a rectangle
width = 10 # Set the width
height = 5 # Set the height
area = width * height
# Display the area
Now, let’s test your understanding. What will the code above print when it’s run? And what is the purpose of the lines that start with #?
Answer: The code will print 50, which is the area of the rectangle (10 * 5). Purpose: The code will print 50, which is the area of the rectangle (10 * 5).
Variables and Data Types
Let’s delve into the world of variables and data types in A-shell. These are fundamental concepts that will empower you to write more versatile and robust code. Think of variables as labeled containers where you can store different kinds of information. It’s like having labeled jars in your kitchen for various ingredients. You can put sugar in a jar labeled “Sugar,” salt in a jar labeled “Salt,” and so on. Similarly, in A-shell, you can create variables with names like name, age, or price to store different pieces of data. Data types are like the labels on those jars, specifying what kind of ingredient each jar can hold. Some common data types in A-shell include: String: This is for storing text, like words or sentences. It’s like a jar labeled “Spices” that can hold various spices. Integer: This is for storing whole numbers, like 1, 2, 3, etc. Think of it as a jar labeled “Grains” that can hold different types of grains. Real: This is for storing numbers with decimal points, like 3.14, 2.5, etc. It’s like a jar labeled “Liquids” that can hold different liquids. Boolean: This is for storing true or false values. It’s like a jar labeled “Flavorings” that can hold either “Sweet” or “Savory.” In A-shell, you don’t need to explicitly declare the data type of a variable. A-shell is smart enough to figure it out based on the value you assign to it. For example: name = “Alice” # This is a string variable age = 30 # This is an integer variable price = 9.99 # This is a real variable is_adult = true # This is a boolean variable Now, let’s see if you’re keeping up. What data type would A-shell assign to the variable quantity in the following code? quantity = 12.5 Answer: Real
Control Flow Statements
Let’s embark on an exciting journey into the realm of control flow statements in A-shell.
These are like the traffic signals of your code, guiding the order in which instructions are executed. They empower you to create logic that makes your Sage X3 customizations dynamic and responsive. Think of it like a choose-your-own-adventure book. Control flow statements allow your code to take different paths based on certain conditions, just like you might choose different storylines based on the decisions you make in the book. Here are some essential types of control flow statements in A-shell: Conditional Statements (If-Then-Else):
These statements allow you to execute certain blocks of code only if a specific condition is met. It’s like saying, “If it’s raining, then take an umbrella; otherwise, don’t.”
Let’s illustrate with a simple example using an If statement:
age = 20
if age >= 18 then
print “You are an adult.”
else
print “You are a minor.”
end if
In this code, the if statement checks if the value of the variable age is greater than or equal to 18. If it is, the code inside the then block is executed, and “You are an adult” is printed. Otherwise, the code inside the else block is executed, and “You are a minor” is printed.
Now, let’s test your understanding. What would happen if the value of age was 15 in the code above? Would it print “You are an adult” or “You are a minor”? And why?
Answer :
It would print “You are a minor” because the age is less than and equal to 18
Loops
Loops are powerful tools in A-shell that allow you to automate repetitive tasks and make your code more efficient. They’re like having a chef in your kitchen who can chop vegetables or stir sauces tirelessly while you focus on the more creative aspects of cooking.
There are two main types of loops in A-shell: For loops and While loops.
For Loops: These loops are used when you know exactly how many times you want to repeat a block of code. It’s like saying, “Chop 10 onions” – you have a specific number in mind.
Here’s a basic example of a For loop:
for i = 1 to 5 step 1 do
print “Number: ” + i
end for
In this code, the loop starts with i equal to 1 and repeats until i reaches 5, incrementing i by 1 each time (step 1). Inside the loop, the print command displays the current value of i. So, this code would print “Number: 1”, “Number: 2”, “Number: 3”, “Number: 4”, and “Number: 5”.
While Loops: These loops are used when you want to repeat a block of code until a certain condition is met. It’s like saying, “Keep stirring the sauce until it thickens” – you don’t know exactly how many times you’ll need to stir, but you’ll keep going until the desired consistency is achieved.
Here’s a basic example of a While loop:
count = 0
while count < 10 do
count = count + 1
print “Count: ” + count
end while
In this code, the loop continues as long as the value of the count is less than 10. Inside the loop, the count is incremented by 1, and the current value of count is printed. This code would print “Count: 1”, “Count: 2”, and so on, until “Count: 10”.
In our next blog, we will discuss Sage X3 Objects and Functions.
In our previous blog we discussed Sage X3 Development Environment.
Explore our YouTube channel for the latest videos and access free training videos for Sage X3.
As registered Sage X3 business partners and expert developers, we have extensive experience in designing and implementing complex workflow solutions.
Contact us today for a consultation. We can help you unlock the full potential of Sage X3 Development, streamlining your operations and driving business growth. Don’t let manual processes hold you back. Let our expertise in Sage X3 development help you optimize your business. The first task is free on us. Terms and conditions apply.